在BIMBase中,对象的编辑有以下几种方法:
1)右键菜单及编辑菜单栏中的移动、复制、镜像、删除功能。对于用户自建对象,平台实现了默认的移动、复制、删除功能,当有特殊需求时(比如删除墙时,同时删除上面的门、窗)需用户代码实现;
2)BPObject
类、BPModel
类均提供删除接口;
3)夹点拖拽功能,用户自建对象需代码实现;
4)属性编辑功能,用户自建对象需代码实现;
3.2.1 移动
移动功能同3.2.2 复制功能,可重写类BPToolMove
实现。
3.2.2 复制
对于自建对象,当软件中的复制功能满足需求时,用户不必再自己实现,若对某自建对象需实现自己的复制功能,可通过重写BPToolCopy
类实现,BPToolCopy
类提供的接口如表3-1所示。
表3-1 BPToolCopy类接口
方法 | 描述 |
---|---|
elementsSelected(List |
过滤选择对象接口 |
dynamic(List |
动态预览接口 |
copy(List |
实现复制效果接口 |
cacheDynamic() | 是否使用默认动态效果 |
[BPExternalToolAttribute(className = "CubeDemo")]
public class CubeCopy : BPToolCopy
{
public override void copy(List<BPObject> elementlist,GeTransform lst)
{
MessageBox.Show ("Cube Copy ! ");
}
}
代码3-7 立方体复制
在示例代码3-7中,通过特性BPExternalToolAttribute定义复制的对象,重写copy接口,实现弹出对话框。在BIMBase复制Cube,则在复制构件的同时,弹出“Cube Copy!”对话框。
3.2.3 镜像
对于自建对象,没有默认的镜像功能,可重写类BPToolMirror
实现。
3.2.4 删除
删除功能同复制功能,可重写类BPToolDelete
实现。
3.2.5 夹点
夹点功能可实现构件的自由拖拽,对于用户自建对象,可按需要实现夹点功能。通过构件类中重写BPGraphicElemen
t的createGripPoints()接口实现夹点的创建,重写dragGripPoints()实现夹点的拖拽编辑。
如示例代码3-8所示,在范例Cube
类中实现夹点功能,在Cube
的每个面的中心创建夹点,拖拽夹点可实现Cube
大小的变化。
protected override List<GePoint3d> _createGripPoints()
{
GeTransform transform = placement.toTransform();
List<GePoint3d> listPt = new List<GePoint3d>();
listPt.Add(GePoint3d.createByTransform(transform, new GePoint3d( mLength / 2, mWidth / 2, 0)));
listPt.Add(GePoint3d.createByTransform(transform, new GePoint3d( mLength / 2, mWidth / 2, mHeight)));
listPt.Add(GePoint3d.createByTransform(transform, new GePoint3d( mLength / 2, mWidth, mHeight / 2)));
listPt.Add(GePoint3d.createByTransform(transform, new GePoint3d( mLength / 2, mWidth, mHeight / 2)));
listPt.Add(GePoint3d.createByTransform(transform, new GePoint3d( 0, mWidth / 2, mHeight / 2)));
listPt.Add(GePoint3d.createByTransform(transform, new GePoint3d( mLength, mWidth / 2, mHeight / 2)));
return listPt;
}
protected override void _dragGripPoints(uint index,GePoint3d grippt, GePoint3d msPos)
{
GeTransform transform = placement.toTransform();
GeTransform trans = GeTransform.createIdentityMatrix();
switch (index)
{
case 0:
mHeight = mHeight + (int)(grippt.z - msPos.z);
trans = GeTransform.create(new GePoint3d(0,0, msPos.z - grippt.z));
placement.fromTransform(GeTransform.createByProduct(trans,transform));
break;
case 1:
mHeight = mHeight + (int)(msPos.z - grippt.z);
break;
case 2:
mWidth = mWidth + (int)(grippt.y - msPos.y);
trans = GeTransform.create(new GePoint3d( 0, msPos.y - grippt.y, 0));
placement.fromTransform(GeTransform.createByProduct(trans,transform)) ;
break;
case 3:
mWidth = mWidth + (int)(msPos.y - grippt.y);
break;
case 4:
mLength = mLength + (int)(grippt.x - msPos.x);
trans = GeTransform.create(new GePoint3d(msPos.x - grippt.x,0,0));
placement.fromTransform(GeTransform.createByProduct(trans,transform));
break;
case 5:
mLength = mLength + (int)(msPos.x - grippt.x);
break;
}
}
代码3-8 立方体夹点实现
3.2.6 属性
对于自建对象,用户可通过重写BPToolProperty
类实现自建对象的属性功能,如图3-4所示,在属性栏显示Cube
的属性长度、宽度、高度,并可通过修改属性值驱动对象变化。
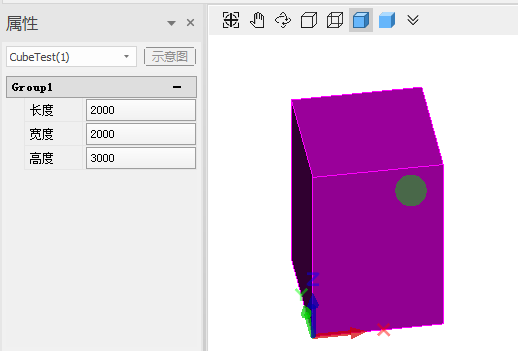
图3-4 Cube属性展示
示例代码3-9展示了Cube
属性实现的部分代码,其中onPropertyGet
中实现从对象获取属性显示在属性栏的功能,onPropertySet
实现在属性栏修改属性,驱动对象修改的功能。
[BPExternalToolAttribute(className = "CubeDemo")]
public class CubePropertyDemo : BPToolProperty
{
enum enCubeProperty
{
Length,
CubePropertyCunt
}
public override void onPropertyGet(List<BPObject> elementlist, BPBimUIPropertyList lst)
{
BPBimUIPropertyItem[] properties = new BPBimUIPropertyItem[(int)enCubeProperty.CubePropertyCunt];
lst.appendGroup("Group1", true);
for (int i = 0; i < elementlist.Count(); i++)
{
CubeDemo cube = (CubeDemo)elementlist.ElementAt(i);
if (0 == i)
{
BPBimUIPropertyItem item =new BPBimUIPropertyItem("长度", cube.length, (IntPtr)0);
properties[(int)enCubeProperty.Length] = item;
}
else
{
BPBimUIPropertyItem oldItem =
properties[(int)enCubeProperty.Length];
if (!oldItem.multiValue())
{
int oldValue = 0;
oldItem.getValue(ref oldValue); if (oldValue != cube.length)
properties[(int)enCubeProperty.Length].setMultiValue(true);
}
}
}
for (uint i = 0; i < properties.Length; i++)
{
lst.append(i, properties.ElementAt((int)i));
}
return;
}
public override bool onPropertySet(List<BPObject> elementlist, int index, BPBimUIPropertyItem item)
{
BPDocument doc = BPApplication.singleton().activeDocument;
for (int i = 0; i < elementlist.Count(); i++)
{
CubeDemo cube = (CubeDemo)elementlist.ElementAt(i);
switch (index)
{
case (int)enCubeProperty.Length:
{
int _value = 0;
if (!item.getValue(ref _value))
return false;
cube.length = _value;
break;
}
default:
break;
}
cube.replaceInDocument(doc);
}
return true;
}
}
代码3-8 立方体属性实现部分代码